Introduction
In decentralized applications, users typically need a crypto wallet to interact with the blockchain. To enable this, developers working on the frontend layer must integrate their application with users' wallet applications. This article is intended for developers using the React library who want to create user interfaces with the popular browser plugin, MetaMask. Here is a step-by-step guide on how to integrate a React application with MetaMask.
A basic application in React
The source code of the application written for this tutarial can be found at this link. I used the following libraries to create the application:
To work with this tutorial, you can use the application provided at the link above, or you can configure the application yourself in React with the help of, for example, create-react-app or vite.
Once our application is configured, you need to make sure that we have all the necessary dependencies installed, to do this, run the command
npm install wagmi ethers
To prepare the application, I also used a component library called Material-ui, if you also want to use it, install the following packages with the command:
npm install @mui/material @emotion/react @emotion/styled
Once the configuration is complete and all the necessary dependencies are installed, we can move on to the next point.
Wagmi Library
To integrate with the MetaMask wallet application, we will use a dedicated library for React called wagmi that contains a sizable number of hooks and functions needed for daily blockchain interactions in frontend applications.
The first step will be to configure the library, to do this we need to wrap our application in a WagmiConfig component passing a client variable with our configuration:
import { WagmiConfig, createClient } from "wagmi";
import { getDefaultProvider } from "ethers";
import { Home } from "./pages";
import "./styles.css";
const client = createClient({
autoConnect: true,
provider: getDefaultProvider()
});
export default function App() {
return (
<WagmiConfig client={client}>
<Home />
</WagmiConfig>
);
}
All available configuration options can be found at this link in the official wagmi documentation
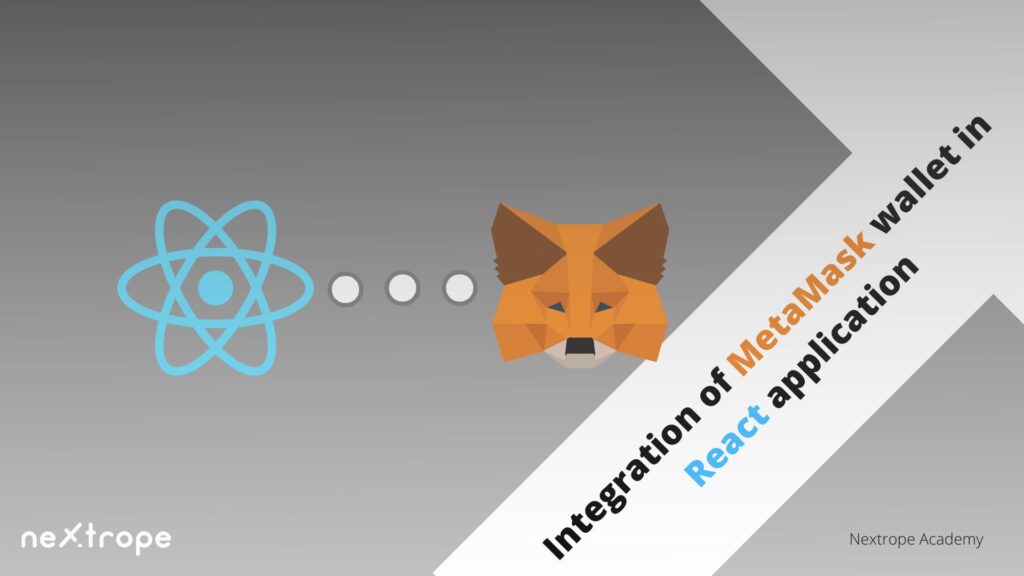
Connecting the MetaMask wallet
Once we have completed the configuration of the wagmi library, we can move on to creating the component responsible for connecting to our wallet. The hooks available in the blibliothek will be helpful in the implementation.
To access the function that will allow us to make a request to connect the wallet, use the useConnect() hooka. To indicate that the wallet we want to connect to is MetaMask, pass the created instance of the InjectedConnector class in the configuration object to the hooka under the connecter key
import { useConnect } from "wagmi";
import { InjectedConnector } from "wagmi/dist/connectors/injected";
...
const { connect } = useConnect({
connector: new InjectedConnector()
});
...
Hook returns us a connect function that we can call, for example, when the button is clicked.
...
<Button onClick={() => connect()}>Connect</Button>
...
To get information about the connected wallet or its connection status, you can use the useAccount() hookup, which returns us such information as:
- the address of the connected wallet
- whether the wallet connection action is in progress
- whether the user's wallet is currently connected in the application
...
const { address, isConnected, isConnecting } = useAccount();
...
If the user of our application managed to connect the wallet we should also allow him to disconnect it, for this we need to use the disconnect function, which we can access with the help of the hookup useDisconnect()
...
const { disconnect } = useDisconnect();
...
With the help of these three simple hooks, we are able to handle wallet connection. The full source code of the component that handles the connection from the sample application:
import { useConnect, useDisconnect, useAccount } from "wagmi";
import { InjectedConnector } from "wagmi/dist/connectors/injected";
import { Card, Button, Heading } from "../../components";
import Typography from "@mui/material/Typography";
import { WalletInfo } from "./WalletInfo";
export const WalletConnect = () => {
const { isConnected, isConnecting } = useAccount();
const { connect } = useConnect({
connector: new InjectedConnector()
});
const { disconnect } = useDisconnect();
return (
<Card>
<Heading sx={{ mb: 2 }}>
{isConnected ? "Your connected wallet:" : "Connect your MetaMask"}
</Heading>
{isConnecting && <Typography>Connecting...</Typography>}
{isConnected ? (
<>
<WalletInfo />
<Button sx={{ mt: 2 }} onClick={() => disconnect()}>
Disconnect
</Button>
</>
) : (
<Button
disabled={isConnecting}
sx={{ mt: 2 }}
onClick={() => connect()}
>
Connect
</Button>
)}
</Card>
);
};
In the above example there is a <WalletInfo /> component, which we will use to display information about the connected wallet, we will deal with its creation in the next step.
Displaying information about the connected wallet
The next step will display to the user information about the connected wallet such as:
- Wallet address
- The current ETH balance of the wallet
To do this, we will prepare two simple components <WalletAddress/> and <WalletBalance/> and , which we will then put into the <WalletInfo/> component:
The address of the currently connected wallet
import { WalletAddress } from "./WalletAddress";
import { WalletBalance } from "./WalletBalance";
export const WalletInfo = () => {
return (
<div>
<WalletAddress />
<WalletBalance />
</div>
);
};
import { useAccount } from "wagmi";
import Typography from "@mui/material/Typography";
export const WalletAddress = () => {
const { address } = useAccount();
return (
<Typography>
<strong>Address: </strong>
{address}
</Typography>
);
};
In order to display the connected wallet, we will use the previously mentioned useAccount() hookup, which returns us the address variable. The implementation of a simple component to display the address looks like this:
import { useAccount } from "wagmi";
import Typography from "@mui/material/Typography";
export const WalletAddress = () => {
const { address } = useAccount();
return (
<Typography>
<strong>Address: </strong>
{address}
</Typography>
);
};
Balance of the currently connected wallet
The wagmi library also has a useBalance() hook, which will make the process of retrieving the current wallet balance much easier for us. The process of fetching this value from the blockchain is asynchronous, so this hook returns on, among other things, such information in variables as:
- isLoading - whether the balance download is in progress
- isFetched - whether the wallet's balance has been downloaded
- isError - whether an error occurred while downloading the data
- data - object containing such fields as:
- value - user balance in WEI units
- formatted - user's balance formatted to ETH units
- symbol - Symbol of the asset for which the balance was downloaded
- decimals - the number of decimal places that the number describing the quantity of the asset can have
For a better understanding of what a WEI, ETH unit or decimals parameter is, I encourage you to read these articles:
- Crypto Denominations Explained: BTC & Satoshis; ETH & Gwei
- Knows “Token Decimals”: When 1 million tokens does not always mean there is only 1 million tokens in total.
To indicate for which wallet we want to retrieve the balance of funds, we need to pass the address of this wallet as a parameter when calling the hookah as follows, to do this we can use the useAccount() hookah again from the previous step:
...
const { address } = useAccount();
const { isLoading, isFetched, isError, data } = useBalance({ address });
...
With this information, we are able to implement an entire component with support for the data loading process:
import { useAccount, useBalance } from "wagmi";
import Typography from "@mui/material/Typography";
import Skeleton from "@mui/material/Skeleton";
export const WalletBalance = () => {
const { address } = useAccount();
const { isLoading, isFetched, isError, data } = useBalance({ address });
return (
<Typography>
{isLoading && <Skeleton width={200} />}
{isFetched && (
<>
<strong>Balance: </strong>
{data?.formatted} {data?.symbol}
</>
)}
{isError && "Fetching balance failed!"}
</Typography>
);
};
Summary
The application presented here is just an example, in production applications developers often have to deal with integrating multiple wallet applications, supporting connectivity on multiple blockchain networks, and interactions of connected wallets with smart contracts. All of these functionalities and much more are supported by the wagmi library presented in this turorial. Therefore, I encourage you to study the documentation of this library to see what capabilities it offers.