In the previous tutorial you learned how to create a smart contract, which is a wallet. In this tutorial we will try to consolidate the knowledge from the previous tutorial, and expand it with new knowledge.
So let's get to work. All the things you may not understand will be explained to you later in the text.
Defining variables
Let's start by defining the variables we'll need to make the smart contract work. We certainly need :
- address of the person to whom the highest bid will be sent,
- time when the auction in question will end,
- address of the person who placed the highest bid,
- why wei is the highest bid,
- a table of addresses and the amount of money they transferred to the smart contract so that those who did not win the auction can withdraw their money,
- variable whether the auction is completed or not.
pragma solidity 0.8.11;
contract Auction {
address payable public beneficiary;
uint public auctionEndTime;
address public highestBidder;
uint public highestBid;
mapping(address => uint) pendingReturns;
bool ended;
}
What can you not understand from the above code?
Mapping in solidity is a key-value array, equivalent to dictionary in other languages.
If you don't know why the auctionEndTime
variable is given in plain uinta, it's because the time when the auction ends will be given in Unix time.
Let's create a constructor that will take the address to which the highest bid will be sent and how long the auction will last.
constructor(
uint biddingTime,
address payable beneficiaryAddress
) {
beneficiary = beneficiaryAddress;
auctionEndTime = block.timestamp + biddingTime;
}
What is worth paying attention to ?
block.timestamp
is a variable that simply means what time it is now given as Unix time of course ;)))
Function for making an offer
Now let's create a function that will be used to submit your bid. The function will return an error if :
- The auction has ended
- Our bid will be lower than the highest bid
At the end of the function execution, the function will emit an event that the highest bid has changed. The frontend application can listen to the emitted events on the smartcontest, so that as soon as the highest bid has changed, it can update it in the user interface.
event HighestBidIncreased(address bidder, uint amount);
event AuctionEnded(address winner, uint amount);
error AuctionAlreadyEnded();
error BidNotHighEnough(uint highestBid);
error AuctionNotYetEnded();
error AuctionEndAlreadyCalled();
function bid() external payable {
if (block.timestamp > auctionEndTime)
revert AuctionAlreadyEnded();
if (msg.value <= highestBid)
revert BidNotHighEnough(highestBid);
if (highestBid != 0) {
pendingReturns[highestBidder] += highestBid;
}
highestBidder = msg.sender;
highestBid = msg.value;
emit HighestBidIncreased(msg.sender, msg.value);
}
As you can see in the code above, we define ourselves events with parameters that we can emit on the blockchain. To emit an event in solidity we type
emit + event name and parameters
We have defined our own errors, which if we want to call them we type in revert + the name of our errror and parameters.
You have probably already guessed that instead of ifs and custom errors we could have used requier.
This function checks if the auction has already ended, if msg.value
is higher than the highest bid, if so we update the mapping pendingReturns so that the person who placed the highest bid earlier can get his money back. We assign the highest bid to msg.value and the highestBidder
to msg.sender
, at the end of the function execution we emit an event that informs that the highest bid has been increased.
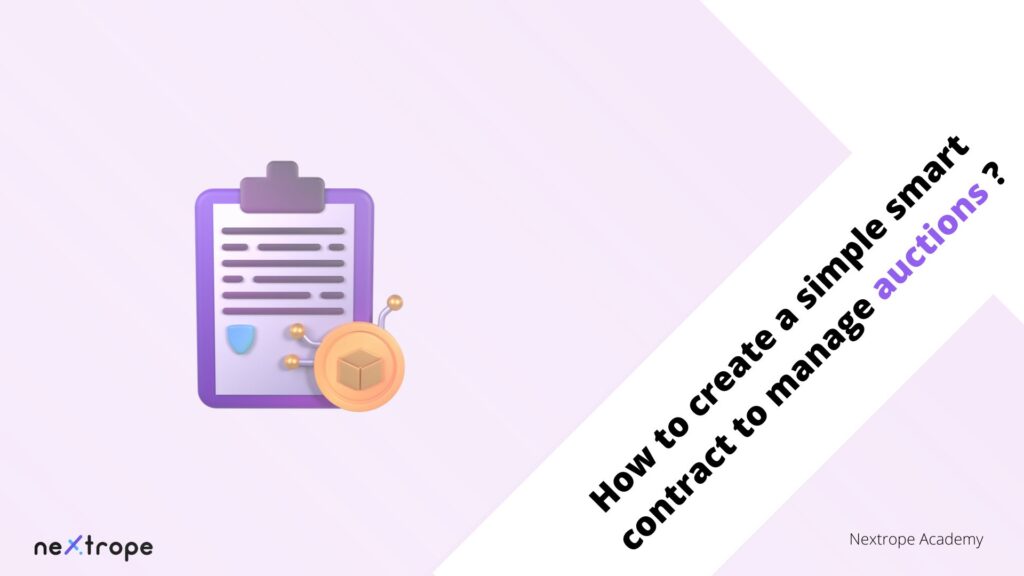
Function to end the auction, and transfer the highest bid to the benefactor
Now let's create a function so that after the auction ends, the beneficiary's address can send money to his wallet.
This function should:
- Return an error if the auction has not yet ended,
- return an error if this function has already been called,
- change the ended variable to true,
- emit an event indicating that the auction has ended,
- transfer an amount of Ethereum equivalent to the highest bid to the benefactor.
function auctionEnd() external {
if (block.timestamp < auctionEndTime)
revert AuctionNotYetEnded();
if (ended)
revert AuctionEndAlreadyCalled();
ended = true;
emit AuctionEnded(highestBidder, highestBid);
beneficiary.transfer(highestBid);
}
Function for people who did not win the auction and want their money back
Now, the last function we need to make our contract ready! It will be for addresses that did not win the auction and want to get their money back. Let's consider what such a function should have in it :
- it should check how much Ethereum you owe in the mapping pendingReturns and assign this value to a variable,
- it should change how much you owe to 0,
- it should send as much Ethereum as you have stored in the variable.
Well, let's get to work !
function withdraw() external{
uint amount = pendingReturns[msg.sender];
pendingReturns[msg.sender] = 0;
payable(msg.sender).transfer(amount);
}
That's the end of today's tutorial ! Our smart contract is ready. In order to practice and consolidate your knowledge, as a task you can try to replace custom errors with requirs. However, if this is not enough for you, you can also improve this contract so that it can be used to conduct several auctions at once.